Project Code
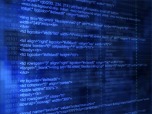
/*
SpoolTech:
Kyle Mathison, Heather Benoit, Bernie Nelson, Chad Hossier, Loria Wang
17 March 2008
MagnosTS v3.14159b Goblin Edition
*/
//pin assignments
int dividerPin = 1; // output pin to divider motor
int lowerSwitchPin = 2; // input pin from the bottom switch
int buttonPin = 8; // input pin from the starter button
int upperSwitchPin = 0; // input pin from the top switch
int greenLED = 12, redLED = 11; // LED output pins
int hPinInput = 4; // H-Bridge PWM input
int hPinFive = 3; // Hbridge input
//global variables
int pushButton, upperSwitch, lowerSwitch = LOW; // initial values
int delayTime = 500; //default delay time
//prototypes
void buttonCheck();
void lowerDivider();
void liftPlatform();
void lowerPlatform();
void setup()
{
//output pins
pinMode(agitatorPin, OUTPUT);
pinMode(greenLED, OUTPUT);
pinMode(redLED, OUTPUT);
pinMode(hPinInput, OUTPUT);
//input pins
pinMode(buttonPin, INPUT);
pinMode(lowerSwitchPin, INPUT);
pinMode(upperSwitchPin, INPUT);
}
void loop()
{
buttonCheck();
lowerDivider();
liftPlatform();
lowerPlatform();
}
/************************************************************************
Function buttonCheck() keeps the green LED on and the red LED off while
checking for a button press. After pressed, the while loop is bypassed,
the red LED comes on, the green LED goes off, and the rest of loop() executes.
************************************************************************/
void buttonCheck()
{
while( pushButton == LOW )
{
digitalWrite(greenLED, HIGH);
digitalWrite(redLED, LOW);
pushButton = digitalRead(buttonPin);
}
digitalWrite(greenLED, LOW);
digitalWrite(redLED, HIGH);
}
/************************************************************************
liftPlatform() raises the polycarbonate platform until 500ms after the
upper switch is tripped.
************************************************************************/
void liftPlatform()
{
// upperSwitch = digitalRead(upperSwitchPin);
digitalWrite(hPinInput, LOW);
analogWrite(hPinFive, 255);
while( upperSwitch == LOW )
{
upperSwitch = digitalRead(upperSwitchPin);
}
digitalWrite(agitatorPin, HIGH);
delay(delayTime);
analogWrite(hPinFive, 0);
//ssdelay(15000);
digitalWrite(agitatorPin, LOW);
}
/************************************************************************
lowerPlatform() does the mirror opposite of what liftPlatform() does.
************************************************************************/
void lowerPlatform()
{
while( pushButton == HIGH )
pushButton = digitalRead(buttonPin);
digitalWrite(hPinInput, HIGH);
analogWrite(hPinFive, 255);
while( lowerSwitch == LOW )
{
lowerSwitch = digitalRead(lowerSwitchPin);
}
delay(delayTime);
analogWrite(hPinFive, 0);
}
/************************************************************************
lowerDivider() lowers the dividers from upright to flat on the platform.
************************************************************************/
void lowerDivider()
{
digitalWrite(dividerPin, HIGH);
delay(delayTime * 4);
digitalWrite(dividerPin, LOW);
}